Looping statements play a crucial role in any programming language. For executing statements repeatedly we use looping statements.
When it comes to Java, two popular loops are the while and do-while loops. Although they both serve similar purposes, they differ in important ways that every Java programmer should understand.
In this article, we’ll explore the difference between the while
and do-while
loop in Java, helping you understand their unique roles and choose the right one for your programs.
Sno. | While Loop | Do-While Loop |
---|---|---|
1 | While loop also called entry-controlled loop. | Do while is an exit-controlled loop. |
2 | Condition is checked at the top of the loop | Condition is checked at the bottom of the loop |
3 | In while loop, condition is checked first then loop body execute. | In do-while, Loop body executes first then condition is checked. |
4 | If the condition is false inititially then loop body will not execute | Always executes at least once, even if the condition is false initially. |
5 | Syntax: while (condition) { // code } | Syntax: do { // code } while (condition); |
6 | In while loop, no semicolon(;) after the while(condition) | In do-while, semicolon(;) is required after the while(condition); |
7 | while loop are also called pre-test loop. | do while is a post-test loop. |
8 | The while loop may or may not execute. | do-while loop will execute at least once. |
9 | Braces are optional if there is only one statement inside the loop. | Braces are required even if there is only one statement inside the loop. |
10 | Use when you want the loop to run only if the condition is true. | Use when you need the loop to run at least once regardless of the condition. |
11 | Typically used in scenarios where the number of iterations is unknown. | Ideal for situations where at least one iteration is required, such as in menus or user prompts. |
What is a while loop in Java?
while loop is also called an entry-controlled looping statement. In while loop first condition is checked, if the condition is true then loop body will be executed otherwise skip the body loop and the cursor will move to the next execution statement in the program.
Syntax:
while (condition) {
// Code to be executed repeatedly
}
Flowchart:
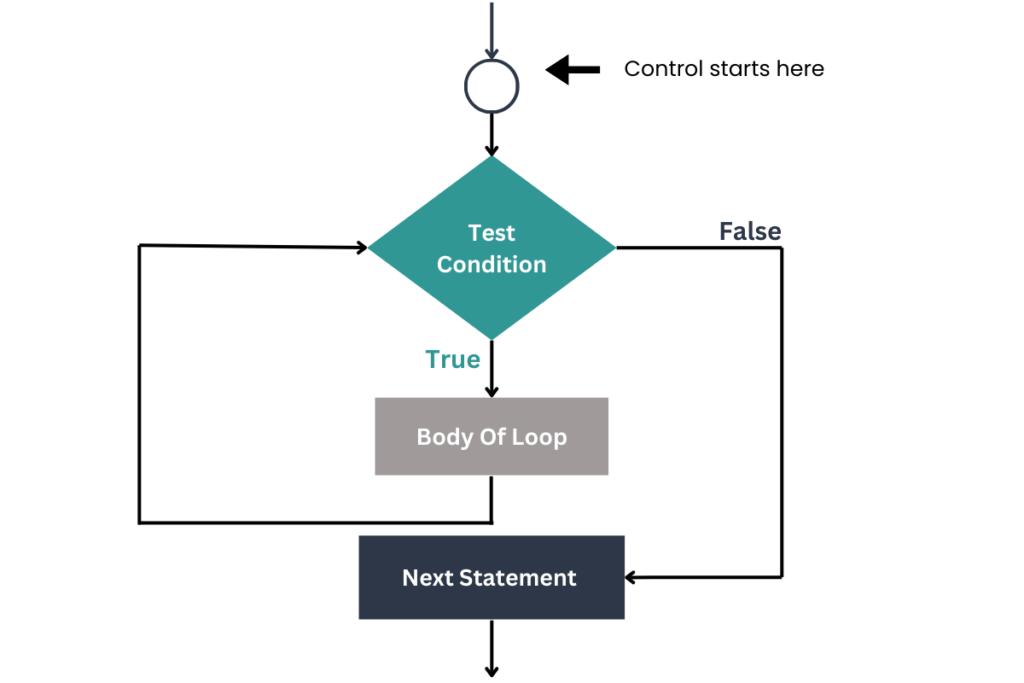
Flowchart Explanation:
- First, control starts from the top and checks the condition.
- If the condition is false then it skips the loop body and the control moves to the next statement.
- If the condition is true the loop body will be executed until the condition become false.
- After loop body execution when the condition becomes false then control moves out from the loop and moves to the next statement.
While loop Sample program:
public class WhileLoopExample {
public static void main(String[] args) {
int counter = 1; // Initialization
while (counter <= 5) { // Condition
System.out.println("Counter is: " + counter); // Loop Body
counter++; // Increment
}
}
}
Program Explanation:
- Initialization:
int counter = 1;
sets the starting value ofcounter
to 1. - Condition:
while (counter <= 5)
checks ifcounter
is less than or equal to 5. If true, the loop body executes. - Loop Body:
System.out.println("Counter is: " + counter);
prints the current value ofcounter
. - Increment:
counter++;
increases the value ofcounter
by 1 on each iteration.
Behavior: The loop prints the value of counter
from 1 to 5. When counter
becomes 6, the condition counter <= 5
is false, so the loop stops.
What is a do-while loop in Java?
do-while loop also called an exit-controlled looping statement. In do-while condition is checked at the bottom. The body loop executes first and then after the condition is checked in the do-while statement. The body loop executes at least once even the condition is false.
Syntax:
do {
// Code to be executed
} while (condition);
Flowchart:
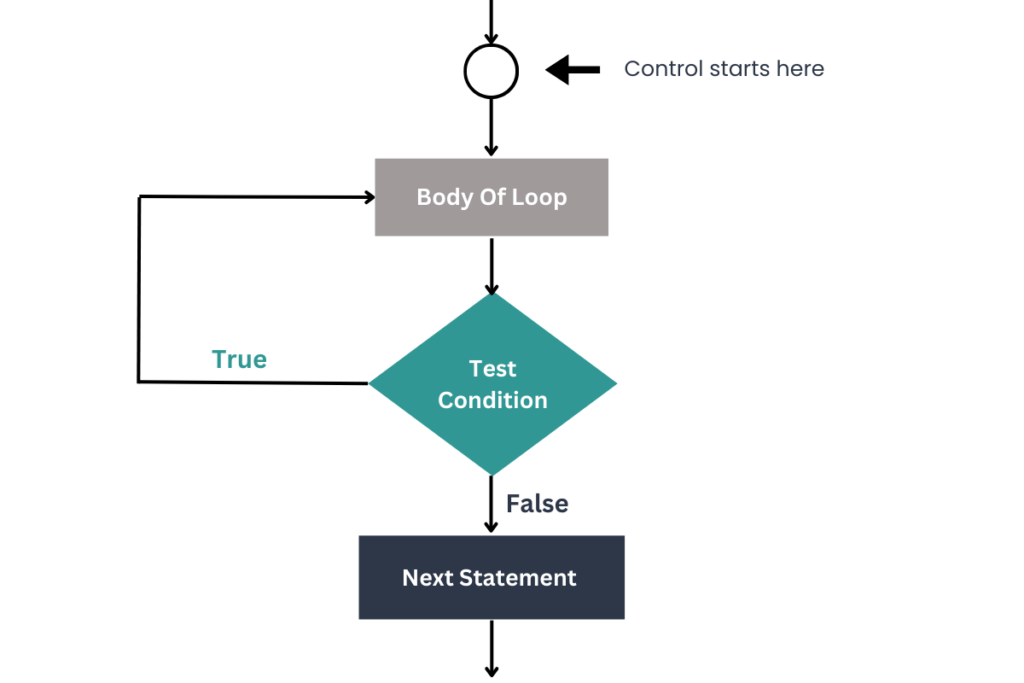
Flowchart Explanation:
- In this, First Body of the loop is executed.
- After body loop execution, the condition is checked.
- If the condition is false then control skip the loop body and jump to the next statement.
- If the condition is true then the loop body will be executed again until the condition becomes false.
Do While Loop Sample Program
public class DoWhileLoopExample {
public static void main(String[] args) {
int counter = 1; // Initialization
do {
System.out.println("Counter is: " + counter); // Loop Body
counter++; // Increment
} while (counter <= 5); // Condition
}
}
Program Explanation:
- Initialization:
int counter = 1;
sets the starting value ofcounter
to 1. - Loop Body:
System.out.println("Counter is: " + counter);
prints the current value ofcounter
. - Increment:
counter++;
increases the value ofcounter
by 1 on each iteration. - Condition:
while (counter <= 5);
checks ifcounter
is less than or equal to 5. After the loop body executes, this condition is evaluated to determine if the loop should continue.
Behavior: The do-while
loop guarantees that the loop body executes at least once. It prints the value of counter
from 1 to 5. After counter
exceeds 5, the loop stops because the condition counter <= 5
becomes false.
Conclusion
In summary, while
and do-while
loops are both used to repeat code, but they differ in when they check their conditions. The while
loop checks the condition before executing the loop body, which means it might not run if the condition is initially false. The do-while
loop, however, ensures the loop body runs at least once by checking the condition after executing the body. Choose the loop based on whether you need the code to run before checking the condition or not.
We’d Love to Hear From You!
Do you have any questions or insights about the differences between while
and do-while
loops? Have you encountered situations where one loop type was clearly more suitable than the other? Share your thoughts and experiences in the comments below! We’re eager to hear how you’ve used these loops in your own Java projects and any tips you might have for others.