A for loop in Java is a looping statement that executes a block of code repeatedly for a specified number of times.
For loop is a popular and convenient loop. The for loop is used in situations where the coder or programmer knows in advance how many times the statement will be executed.
Unlike while and do while, variable initialization, condition checking, and increment or decrement operations can all be done in the same statement in a for loop.
This means, 3 loop control elements (initialization expression, test condition, and increment/decrement expression)
are present in one location(for loop)
.
Syntax
for (initialization; condition; update) {
// Code to be executed
}
Explanation:
- Syntax starts with a
for
keyword. initialization
is used to initialize the loop variable followed by a semicolon (;). The loop variable is the local variable of the for loop. we can not access the loop variable (local variable) outside the for loop.condition
is used to check the test condition followed by a semicolon (;).update
is used for increment and decrement operations.- Three expressions are separated by a semicolon(;) in the for loop header.
- After that {} curly braces are used.
Flowchart
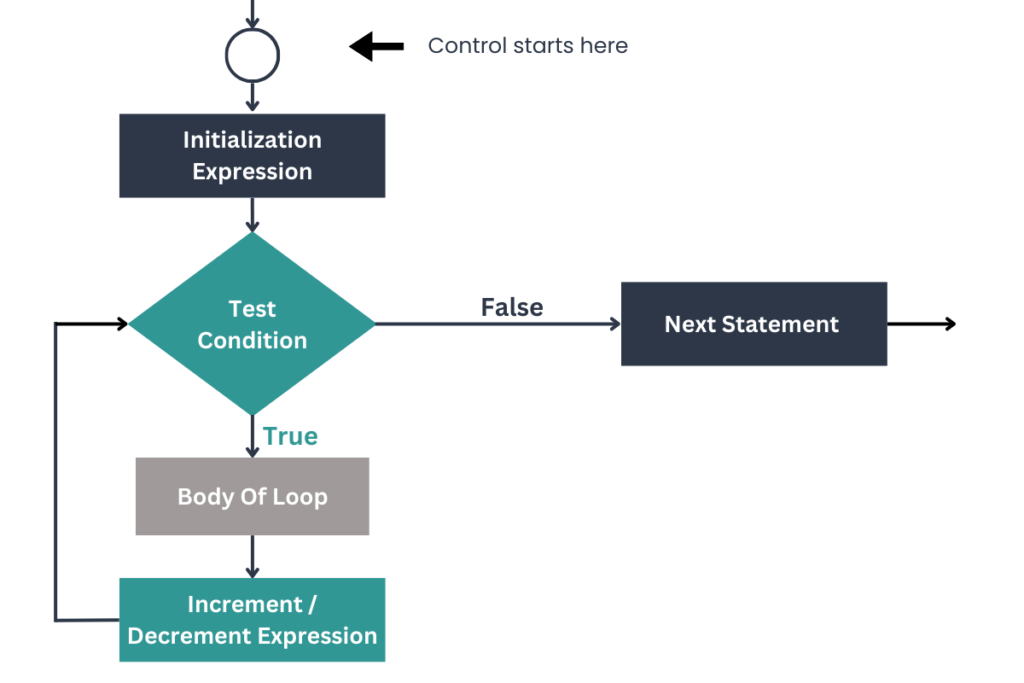
let’s understand the for-loop flowchart with the help of the explanation below:
Explanation:
- The first control starts from the top of the flowchart.
- Then, the control moves to the initialization part, Here variable initialization is done.
- After variable initialization control goes to the test condition part and checks the condition, if the condition is true then the body of the loop (code inside for loop) will be executed.
- When the body of the loop is executed successfully then control goes to the increment/decrement expression. After the increment/decrement expression control goes to the test condition for checking the condition. If the condition is true again then the same process repeats again until the condition becomes false.
- When the condition becomes false then the control skips the loop body and goes to the next statement in the program.
For Loop and Its Optional Parts in Java
All three expressions (initialization expression, test condition, and increment/decrement expression)
in the for loop are optional. we can remove these expressions from the for-loop header.
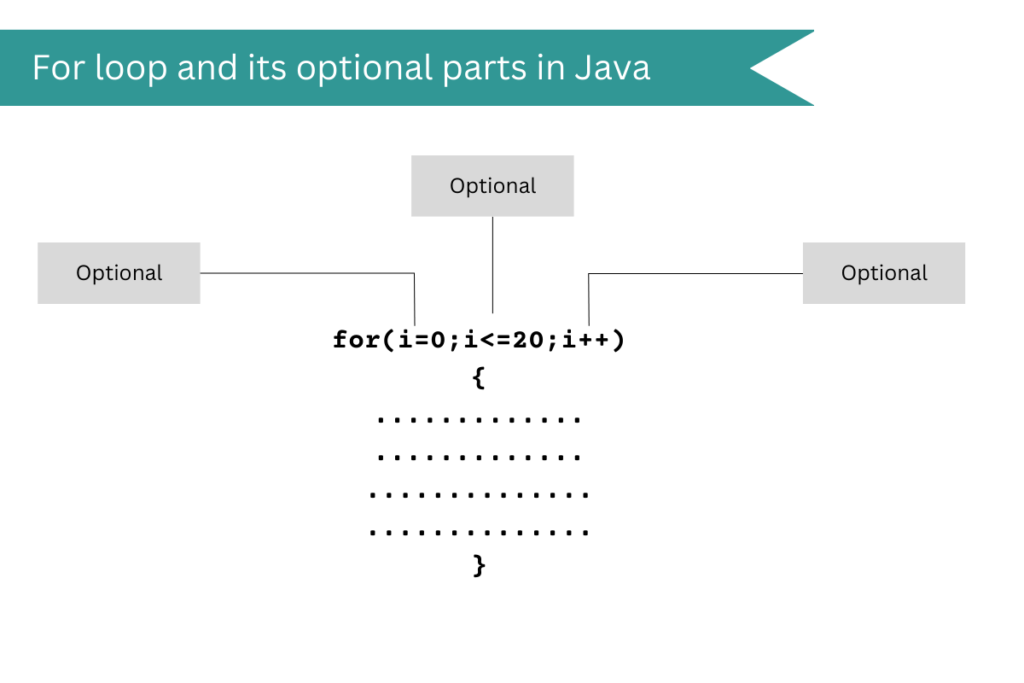
- Initialization expression (for-loop local variable) can be removed if the variable has already been initialized earlier in the program.
- If the test condition is omitted then the for-loop becomes an infinite loop in java. In that case, we use a break statement or throw an exception to stop the loop.
- Increment and decrement expressions can be removed if not required. If it is required then we can place that expression(i++) inside the body loop.
We can write for-loop in Java in different ways without any errors. See example below:
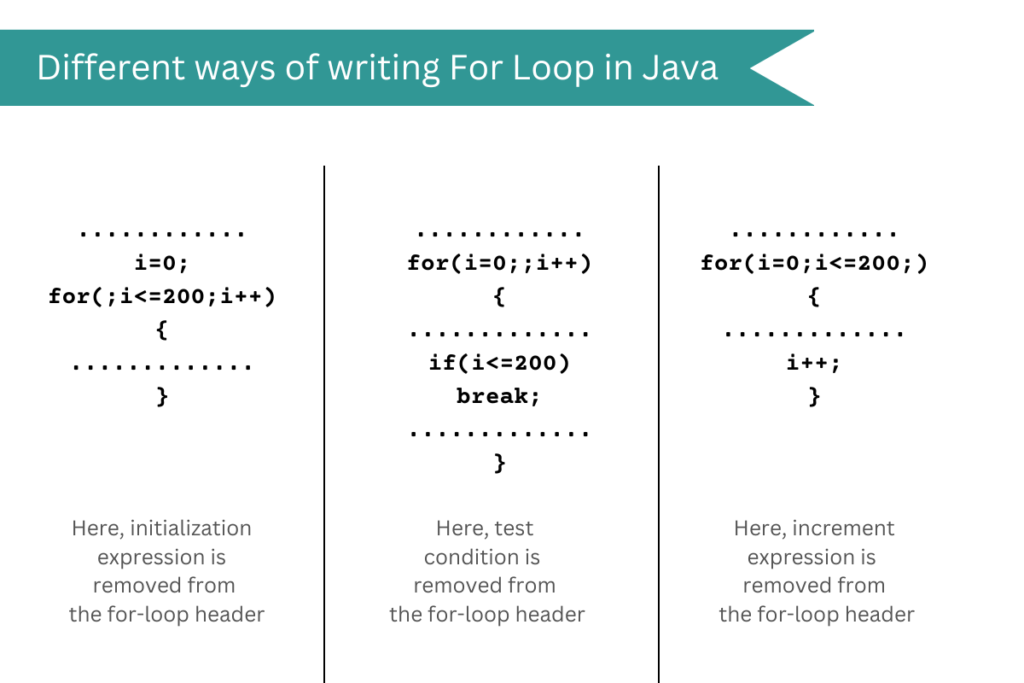
Sample for-loop Program in Java
public class SampleForLoopProgram {
public static void main(String[] args) {
// For loop to print numbers from 1 to 15
for (int j = 1; j <= 15; j++) {
System.out.println("Number is: " + j);
}
}
}
Explanation:
- Our class name is
SampleForLoopProgram
, This is called class declaration. public static void main(String[] args)
is the main method, Execution starts from the main method.- Then control jumps to the for-loop header for(int j=1; j<=15; j++)
- First, the initialization part
int j=1;
value of j is 1. - j is initialized only once then the control moves to the test condition(j<=15) and checks the condition (1<=15), which means true.
- Condition is true then the loop body
System.out.println
statement executes and prints the valueNumber is 1
. - After the loop body execution is complete, the control moves to the update expression
j++
- Increment the value of j now the value of j is 2 and the control moves to the condition expression and checks the condition and the condition is (2<=15) again true, again loop body executes.
- This process repeats until the condition becomes false.
- When the condition becomes false then the loop body will be skipped and the control moves to the next statement in the program if present.
Output:
Number is: 1
Number is: 2
Number is: 3
Number is: 4
Number is: 5
Number is: 6
Number is: 7
Number is: 8
Number is: 9
Number is: 10
Number is: 11
Number is: 12
Number is: 13
Number is: 14
Number is: 15
Program Reverse a string in Java using for loop
public class ReverseStringProgram {
public static void main(String[] args) {
String originalString = "moc.yarhcetia";
String reversedString = "";
// Loop through the string from end to start
for (int j = originalString.length() - 1; j >= 0; j--) {
reversedString += originalString.charAt(j);
}
System.out.println("The Original String is: " + originalString);
System.out.println("The Reversed String is: " + reversedString);
}
}
Explanation:
- In this program we have two variables, one is the
originalString
with the value “moc.yarhcetia" and the
second is thereveresedString
with no value (empty). - In this for loop, variable j is initialized with the value
originalString.length()-1
means 12. length()
is the function, this function gives the length of the string,moc.yarhcetia
string total length is 13.- In string, characters start from 0 index which means m is on 0 index, o is on 1 index, c is on 2 index, and so on, that’s why value 1 is minus from the total length of
originalString.length()-1
means 12. - Now the value of j is 12 after the initialization, the condition is checked and the test condition is (12>=0) which means true.
- Condition true and control moves to the body of the loop.
- In the loop body,
originalString.charAt(j)
evaluated, charAt() is a function that returns the character at the specified index in the string and the value is “a” because the value of j is 12 and on 12 index character “a” is present. - After
originalString.charAt(j)
evaluation, value is assigned to the variablereversedString
with the help of the addition assignment operator (+=). It is used to add a value to a variable and then assign the result back to that variable. - After loop body execution is complete the control moves to the decrement expression j– and decrement the value of j now the value is 11 and the control moves to the condition now the condition is (11>=0) which means true.
- Again loop body executes until the condition becomes false.
- At the end, print the values.
Output:
The Original String is: moc.yarhcetia
The Reversed String is: aitechray.com
If you want to make an infinite loop in Java with the help of a for loop then use the below program
Program infinite for loop in Java
public class InfiniteForLoop{
public static void main(String[] args) {
// Infinite for loop
for (;;) {
System.out.println("Infinite loop statement");
}
}
}
Explanation:
- All expressions in the for loop header are left blank.
- If we omit the condition from the for-loop, then it becomes an infinite loop, running continuously without stopping.
Conclusion
The for
loop in Java is a powerful tool for handling repetitive tasks and working with ranges of values efficiently. Mastering its use will help you write cleaner, more effective code. Practice with different examples and explore additional resources to strengthen your skills and apply them to real-world programming challenges.
We’d Love to Hear From You!
Do you have questions or feedback about the for
loop in Java? We’re here to help! Whether you need further clarification, have suggestions, or just want to share your thoughts, please leave a comment below. Your feedback is invaluable in helping us improve our content and support others. Don’t hesitate to reach out—we’re excited to hear from you!
Related Articles:
While Loop In Java: Syntax, Flowchart, and Practical Examples
do while loop in Java: Syntax, Flowchart, and Practical Examples
Additional Resources
For more information on Java for
loops and other control flow statements, check out the official Java Documentation. It provides clear explanations and examples to help you understand how to use these features effectively.