Foreach Loop is also called Enhanced for loop or Numerical for loop. It is the enhanced version of the traditional for loop. It is easier to write and read than a traditional loop (for, while).
In a foreach
loop, there is no need to handle manual indexing.
A for-each loop is used to access all elements of an array. However, we can not use a for-each loop to access the part of an array or modify the particular value in the array.
Syntax
for (dataType item_var : arrayName) {
// statements to be executed
}
Explanation:
dataType
is the type of the elements of an array or collection, for example : (String, int).item_var
is the iteration variable or local variable of the for-each loop, we cannot access the item_var outside the loop. It is available only within the loop.- item_var variable holds the value that is the same as the current array element.
arrayName
is the array or collection through which to iterate.
How does Foreach work in Java?
for(int j: nums){
System.out.println(j);
}
Explanation:
- In this,
nums
is an array andj
is the loop variable. - On the first iteration, the first value of a nums array which is stored in
nums[0]
is assigned to variable j. - Then print the value j after that second iteration will start.
- In the second iteration, the next value of a nums array which is stored in
nums[1]
is assigned to variable j. - Again print the value of j.
- Repeat the same process until the last element of the array nums is processed.
let’s understand the for-each loop with the help of a flowchart.
Flowchart
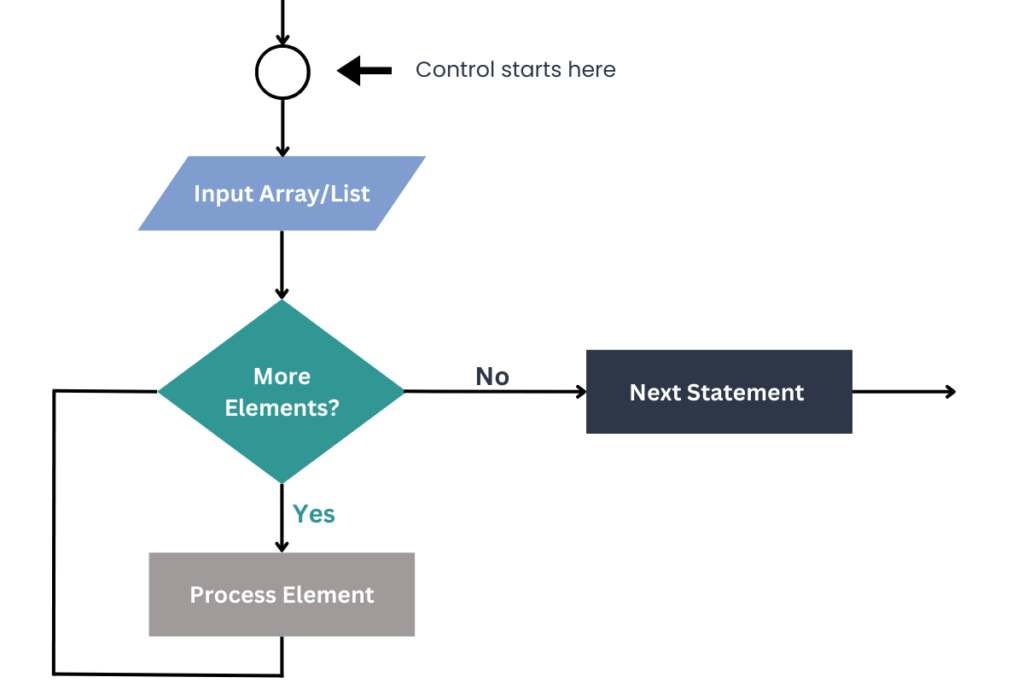
Explanation:
- Control starts from the top.
- Control moves to the
Input Array/List
and initializes the array here. - Then control moves to the loop condition and checks (More Elements?).
- If there is more elements in the array then the
Process Element
block will be executed. - After execution of the process element block, the control moves to the loop condition.
- Again check the elements of an array, Repeat steps 4 and 5 until the last element is processed.
- After the last element is processed again check the
More Elements
condition. - If the element is not present then skip the for-each loop body and control moves to the Next Statement.
Simple Foreach Program
public class SimpleForeachProgram {
public static void main(String[] args) {
int[] numbers = {100, 200, 300, 400, 500};
// foreach loop to print each number in the array
for (int item : numbers) {
System.out.println(item + " is present.");
}
System.out.println("Outside The For Each");
}
}
Explanation:
- The class name is
SimpleForeachProgram
. - Code execution starts from the
main()
method. - Initialize the array numbers with values {100,200,300,400,500}
- Then, start the loop, The first value of the numbers array is assigned to the loop variable
item
and the loop body will be executed (prints the value item with the message) - The first value means 100 and the value 100 is stored in the 0 index means
numbers[0]
store100
, numbers[1] store 200, numbers[2] store 300, and so on. - After processing the last element, the loop body will be skipped. And control moves to the next statement and prints the value
Outside The For Each
.
Output:
100 is present.
200 is present.
300 is present.
400 is present.
500 is present.
Outside The For Each
Conclusion
The foreach
loop in Java provides a simple and efficient way to iterate over arrays and collections without the need for manual index handling. It enhances code readability and reduces the risk of common errors associated with traditional loops. It keeps your code simple and reduces mistakes.
In short, the foreach
loop helps you write cleaner and easier-to-read code, making it perfect for working with arrays and collections in a simple way.
We’d Love to Hear From You!
Do you have questions or feedback about the foreach loop in Java? We’re here to help! Whether you need further clarification, have suggestions, or just want to share your thoughts, please leave a comment below. Your feedback is invaluable in helping us improve our content and support others. Don’t hesitate to reach out—we’re excited to hear from you!
Related Articles:
For loop In Java: Syntax, Flowchart, and Practical Examples
While Loop In Java: Syntax, Flowchart, and Practical Examples
do while loop in Java: Syntax, Flowchart, and Practical Examples
Additional Resources
For more information on Java for-each
loop statement, check out the official Java Documentation. It provides clear explanations and examples to help you understand how to use these features effectively.