In the world of programming, decision-making is crucial. It allows programmers to control the flow of execution within a program. In Java, the if
statement is a fundamental tool for decision-making, enabling the program to execute specific code blocks based on certain conditions.
What is an if
Statement?
if
statement is a control flow statement. If the specified condition is true then only the block of if
statement will be executed.
Suppose the condition is false then a block of if
statement or body of if
statement will not execute and control is transferred to the next statements.
Syntax
if (condition) {
// if the condition is true
// this block will be executed
}
Flowchart
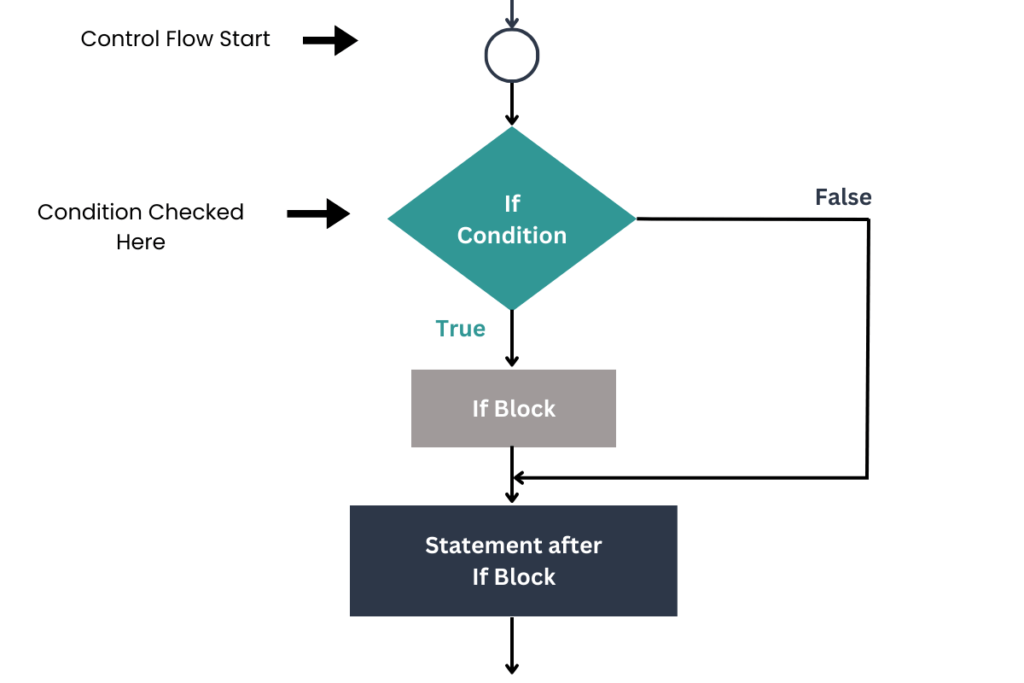
Explanation
Let’s understand if
statement working with the help of a flowchart. First control start and go towards if
condition and then the condition is checked if the condition is false then the body of if means “if
block in the flowchart” will not execute and control transferred to the “Statement after If block in the flowchart”.
Another case is if the condition is true then “if
block in the flowchart” will execute and after execution is complete, control is transferred to the “Statement after If block in the flowchart”.
Types of conditions
Let’s talk about conditions, 3 types of conditions are used with if blocks.
1. Boolean Expression: In this, the condition itself is a boolean value.
boolean isRaining = true;
if (isRaining) {
System.out.println("Yes! It is raining.");
}
In this code, the variable name is isRaining of boolean data type and the value is true. The condition is checked, and the condition is true so this line of code “System.out.println("Yes! It is raining.");
” will be executed and the message “Yes! It is raining.
” print on the console.
Yes! It is raining.
2. Comparison Operators: In this, comparison operators are used in condition.
==
checks for equality.!=
checks for inequality.>
,<
,>=
,<=
compare numerical values.
int age = 20;
if (age >= 18) {
System.out.println("You are an adult.");
}
In this code, if a variable age is greater or equal to the value 18 then the message “You are an adult.” will be printed on the console.
You are an adult.
3. Logical Operators: With the help of logical operators we can combine two conditions in if
statement.
&&
(AND), ||
(OR), !
(NOT)
int score = 75;
if (score >= 70 && score < 80) {
System.out.println("Your grade is B.");
}
In this code, if the score value is between or equal to 70 and 80 then the output will be “Your grade is B.“
Program Example
public class IfStatementExample {
public static void main(String[] args) {
// Example age to check
int age = 16;
// Check if the person is an adult
if (age > 18) {
System.out.println("Person is an adult with age " + age);
}
// Check if the person is a teenager
if (age >= 13 && age <= 18) {
System.out.println("Person is a teenager with age " + age);
}
// Check if the person is younger
if (age < 13) {
System.out.println("Person is younger with age " + age);
}
}
}
Explanation
- Adult Check:
if (age > 18)
checks if the age is greater than 18, and prints that the person is an adult.
- Teenager Check:
if (age >= 13 && age <= 18)
checks if the age is between 13 and 18, inclusive, and prints that the person is a teenager.
- Younger Check:
if (age < 13)
checks if the age is less than 13, and prints that the person is younger.
How It Works
- Adult: The program will print “Person is an adult with age 16” only if the age is greater than 18.
- Teenager: The program will print “Person is a teenager with age 16” if the age is between 13 and 18.
- Younger: The program will print “Person is younger with age 16” if the age is less than 13.
Now the question is what is the output of this program, our variable age is 16 in the program above. so,
age > 18
: The condition is false because16
is not greater than18
.age >= 13 && age <= 18
: The condition is true because16
is between13
and18
, inclusive. Thus, it prints “Person is a teenager with age 16”.age < 13
: The condition is false because16
is not less than13
.
Output
Person is a teenager with age 16
Tip: Using if
Statements Without Curly Braces in Java
In Java, you can technically use if
statements without curly braces {}
when the statement following the if
is a single line. For example:
if (condition)
System.out.println("Fun with java");
Advantages:
- Conciseness: Omitting curly braces can make your code look cleaner and more compact when dealing with single-line statements.
Limitations:
- Readability: Without curly braces, it might not be immediately clear which statements are associated with the
if
condition, especially in more complex code. - Maintenance: Adding additional statements later can lead to bugs. For example, consider the following code:
if (condition)
System.out.println("Fun with java");
System.out.println("This line will always execute.");
Here, only the first println
statement is part of the if
block. The second println
is always executed, which might not be the intended behavior.
Best Practices: For clarity and to avoid accidental errors, it’s often recommended to use curly braces {}
even for single-line statements:
if (condition) {
System.out.println("Fun with java");
}
This practice helps ensure that all intended statements are included in the if
block and makes your code easier to read and maintain.
Conclusion
The if
statement in Java helps your program make decisions. It lets you run certain code only when specific conditions are true. By using if
statements, you can make your Java programs smarter and more flexible. Practice using them to handle different situations in your code. With time, you’ll become more confident and skilled in Java programming!
We’d Love to Hear From You!
Have any questions or feedback about the if
statement in Java? Feel free to leave a comment below! Whether you need further help, have a suggestion, or just want to share your thoughts, we’re here to assist you. Your input is valuable and helps us improve. Don’t hesitate to reach out—we’re excited to hear from you!
Explore More Java Topics
Interested in learning more about Java control structures? Check out our related posts:
- If Else in Java – Dive into the If Else statement and understand its practical uses.
- If Else If in Java – Explore the basics of the If Else If and how to implement it effectively.
Feel free to explore these articles to enhance your understanding of Java programming!
Additional Resources
To deepen your understanding of the If statement in Java, check out the official Java Documentation on Control Flow Statements provided by Oracle. This resource offers a comprehensive overview of Java’s control flow statements, including detailed explanations and examples.