A break
statement is used to terminate or stop loop execution immediately when it is encountered. It can be used in all loops (while, do-while, for, and for-each) and also in switch statements in Java.
When we use a break
statement in any loop or switch statement, it is typically placed within a if
condition or other control logic that determines when it should be executed. Once that condition is met, and the break
statement is encountered, it immediately terminates the loop or the switch case execution and shifts control outside the loop body or the switch statement case.
When the break statement is encountered in any loop then it skips the remaining part of the loop and shifts control outside the loop body.
Syntax:
break;
break keyword is used in Java to terminate the loop executions and switch statements execution.
Flowchart
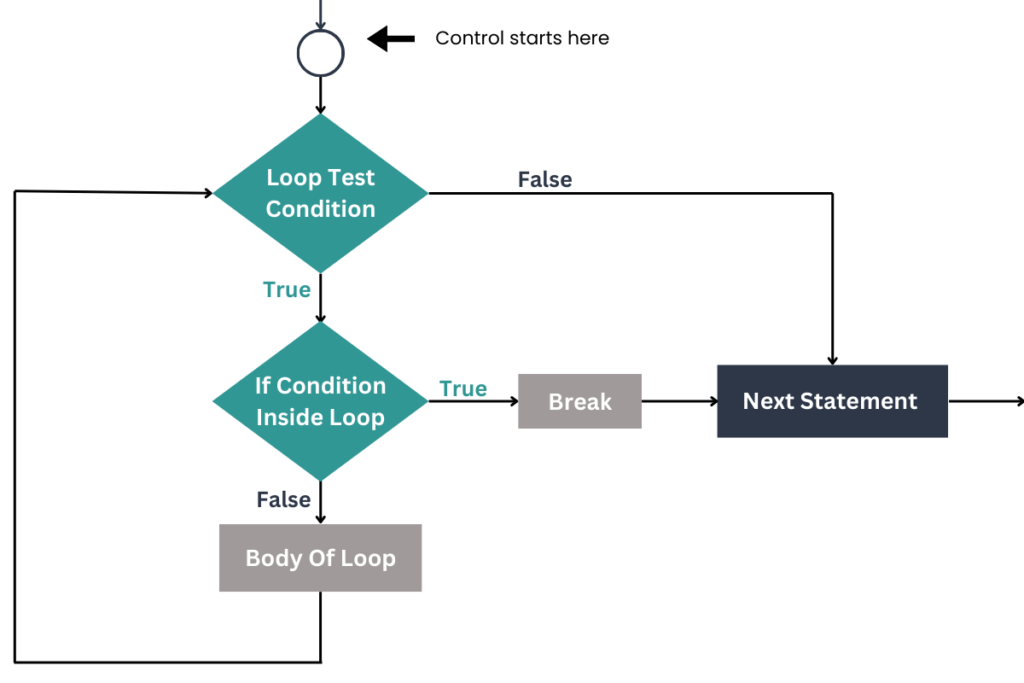
Explanation:
- Inside the loop, we have another
if
condition - If this condition is true then the break statement will be executed and the cursor will move out of the loop body
- Otherwise, the loop body will be executed.
Now we see the working of break statements in all looping statements with the help of programs so let’s start,
Program Example 1: How to use break statement with while loop in Java
public class BreakWithWhile {
public static void main(String[] args) {
int i = 100;
while (i < 1000) {
if (i == 600) {
break; // Terminates the loop when i is 600
}
System.out.println(i);
i = i + 100;
}
System.out.println("While Loop terminated.");
System.out.println("Now i value is " + i);
}
}
Explanation:
- Initialize the variable i with the value 100
- While loop runs until the value of i becomes 1000
- Check the condition (i==600) inside the loop body
- If the condition is true then break statement execute and terminate the loop, shift the control outside the loop body
- Output:
- For the first five iterations when the value of i is(100,200,300,400,500), the loop body
println
statement execute and print the value of i - When the value of i becomes 600 then break execute, terminate loop and shift control outside, and execute the outside
println
statements.
- For the first five iterations when the value of i is(100,200,300,400,500), the loop body
100
200
300
400
500
While Loop terminated.
Now i value is 600
Now we use the same program using do-while
loop but with different condition and see the working of the break statement in do-while
loop. Our mission is just to clarify and understand the concept.
Program Example 2: How to use break statement with a do-while loop in Java
public class BreakWithDoWhile{
public static void main(String[] args) {
int i = 100;
do {
if (i == 700) {
break; // Terminates the loop when i is 700
}
System.out.println(i);
i = i + 100;
} while (i < 1000);
System.out.println("Do-while Loop terminated.");
System.out.println("Now i value is " + i);
}
}
Explanation:
- The variable i is initialized with the value 100
- In do-while, the loop body executes for the first time without checking the condition
- This loop will run until the value of i becomes 1000
- But we specify the condition (i==700) inside the body loop
- When this condition becomes true then the loop will be terminated with the help of a break statement
- Output:
- For the first six iterations when the value of i is(100,200,300,400,500,600), the loop body
println
statement executes and prints the value of i into the console - When the value of i becomes 700 then the break statement executes, terminates the loop and shifts control outside, and executes the outside
println
statements.
- For the first six iterations when the value of i is(100,200,300,400,500,600), the loop body
100
200
300
400
500
600
Do-while Loop terminated.
Now i value is 700
Now we move ahead and use the break statement with the for loop.
Program Example 3: How to use break statement with for loop in Java
public class BreakWithForLoop{
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
System.out.println("Break statement is executed. Now value of i is " + i);
break; // Terminates the loop when i is 5
}
System.out.println(i);
}
System.out.println("For Loop terminated.");
}
}
Explanation:
- In this, we initialize the variable i inside for loop header with the value 1
- This loop will run until i value becomes 10
- But we use specific conditions inside for loop
- On every iteration print the value of i
- When this condition is met, the break statement will execute and print the statement and shift the control outside the loop body.
- At the end “For loop terminated ” statement will be executed.
1
2
3
4
Break statement is executed. Now value of i is 5
For Loop terminated.
Program Example 4: How to use break statement with for each loop in Java
public class BreakWithForEach {
public static void main(String[] args) {
String[] things = {"Table", "Chair", "Bottle", "Glass", "Door", "Box"};
for (String thing : things) {
if (thing.equals("Glass")) {
System.out.println("Break is executed and");
System.out.println("Thing value inside condition is: " + thing);
break; // Terminates the loop when Glass is encountered
}
System.out.println(thing);
}
System.out.println("ForEach Loop terminated.");
}
}
Explanation:
- Now we use an array variable named things and stores some string values inside things variable
- This loop name is enhanced for loop and this will run until all the elements of the specified array (or collection) have been processed.
- And print the value of an array of things
- But we specify the condition, when this condition is met then print some statement and execute the break statement.
- Control is transferred outside the loop and prints the outside statement if present.
Table
Chair
Bottle
Thing value inside condition is: Glass
ForEach Loop terminated.
Program Example 5: How to use break statement with Nested for loop in Java
public class BreakWithNestedLoop{
public static void main(String[] args) {
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (j == 2) {
System.out.println("Inner loop is terminated at j value " + j);
break; // Terminates the inner loop when j is 2
}
System.out.println("value of i = " + i + ", value of j = " + j);
}
System.out.println(); // For a new line between outer loop iterations
}
System.out.println("Nested loop terminated.");
}
}
Explanation:
- Now we are using nested for loop to show the working of break statement. In nested for loop break statements works differently.
- We have two loops one is an outer loop and the second is the inner loop
- We specify the condition inside the inner loop
- When this condition is met then only the inner loop body is terminated not the outer loop body.
- Control is transferred to the outer loop
- This is the normal behavior of a break statement, when the break is encountered then current loop body is skipped or terminated and control is transferred to the outside of the loop
- The outer
for
loop runs withi
values from 1 to 3 - The inner
for
loop runs withj
values from 1 to 3 - When
j == 2
, thebreak
statement terminates the inner loop. Before breaking, a message is printed showing that the inner loop terminates whenj
is 2
value of i = 1, value of j = 1
Inner loop is terminated at j value 2
value of i = 2, value of j = 1
Inner loop is terminated at j value 2
value of i = 3, value of j = 1
Inner loop is terminated at j value 2
Nested loop terminated.
To overcome this limitation we use labeled break statements.
Labeled break in Java
A labeled break statement is used to break out of a specific outer loop or block, not just the innermost loop. It allows you to exit from a nested loop or block based on the label specified.
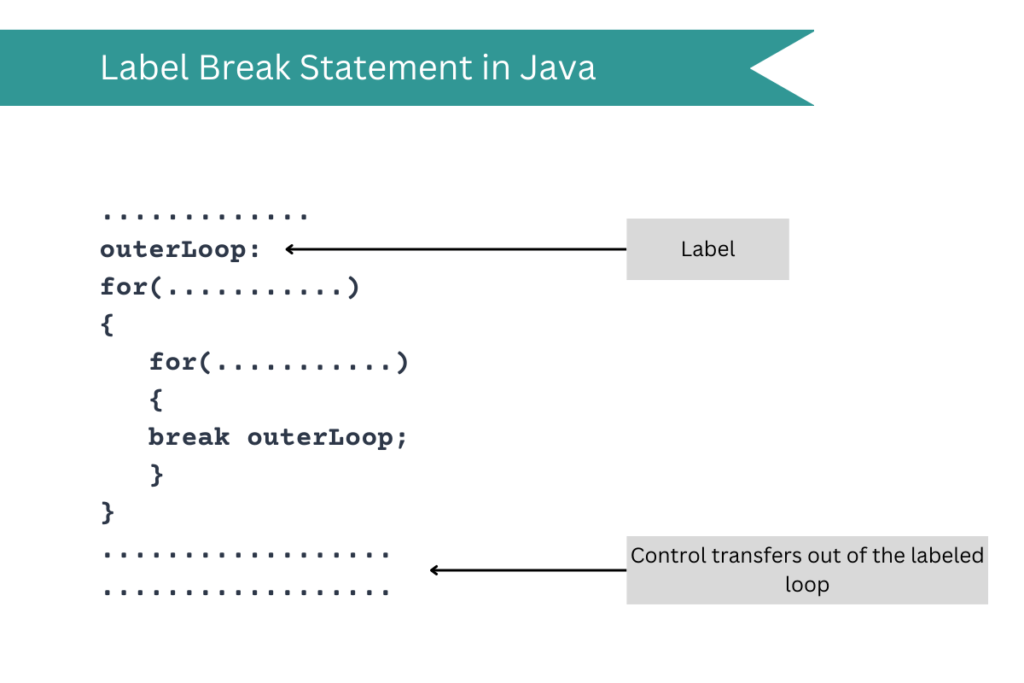
labeled break Syntax
labelName:
for (initialization; condition; update) {
for (initialization; condition; update) {
// some code
if (condition) {
break labelName; // Exits the outer loop with the label
}
}
}
First, you need to write a label on the specific loop or block from which you want to exit. The label name should be followed by a colon (:
).
Then, use the label name with the break
statement to exit from that loop or block.
Now we use labeled break statements with the previous nested loop program.
Program Example 6: How to use labeled break statements in Java
public class LabeledBreakProgram{
public static void main(String[] args) {
outerLoop:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (j == 2) {
System.out.println("Inner loop and Outer Loop both are terminated at j value " + j);
break outerLoop; // Terminates the inner and outer loop when j is 2
}
System.out.println("value of i = " + i + ", value of j = " + j);
}
System.out.println(); // For a new line between outer loop iterations
}
System.out.println("Nested loop terminated.");
}
}
Explanation:
- The program has two loops: an outer loop (
i
) and an inner loop (j
). - The label
outerLoop:
marks the outer loop. - When
j == 2
, thebreak outerLoop;
stops both loops. - The output shows values for
i = 1
andj = 1
. Whenj
reaches 2, both loops exit, and the program ends.
This is how the labeled break works to exit from both loops together.
value of i = 1, value of j = 1
Inner loop and Outer Loop both are terminated at j value 2
Nested loop terminated.
Program Example 7: How to use break statement with Switch in Java
public class Main {
public static void main(String[] args) {
String fruit = "Avocado";
switch (fruit) {
case "Apple":
System.out.println("You chose Apple!");
break;
case "Avocado":
System.out.println("You chose Avocado!");
break;
case "Cherry":
System.out.println("You chose Cherry!");
break;
case "Mango":
System.out.println("You chose Mango!");
break;
default:
System.out.println("Unknown fruit");
}
}
}
Explanation:
- In this program, the
switch
statement evaluates thefruit
variable, which is set to “Avocado”. - It checks each
case
against the value offruit
. - When it finds a match with “Avocado”, it executes the corresponding code block, printing “You chose Avocado!”.
- The
break
statement then exits the switch, preventing any further checks.
You chose Avocado!
Conclusion
In conclusion, the break
statement is a valuable feature in Java that lets you exit loops and switch cases when needed. By mastering its syntax and seeing how it works in flowcharts and examples, you can streamline your code and enhance its efficiency. Whether you’re working with for
, while
, or switch
statements, the break
statement helps keep your programs clear and easy to manage, making your coding experience smoother and more enjoyable!
We’d Love to Hear From You!
Do you have questions or feedback about the break
statement in Java? We’re here to help! Whether you need further clarification, have suggestions, or just want to share your thoughts, please leave a comment below. Your feedback is invaluable in helping us improve our content and support others. Don’t hesitate to reach out—we’re excited to hear from you!
Related Articles:
While Loop In Java: Syntax, Flowchart, and Practical Examples
do while loop in Java: Syntax, Flowchart, and Practical Examples
For loop In Java: Syntax, Flowchart, and Practical Examples
Switch case in Java: Syntax, Flowchart, and Practical Examples