In the real world, a class is like the design or blueprint of a product, and an object is the actual product with specific properties and behavior.
Sno. | Class | Object |
---|---|---|
1 | Class is a template or blueprint or structure of the object | Object is an instance of the class with actual value and perform actions through methods |
2 | Class is created only one time | Objects are created multiple times |
3 | No memory is allocated for instance variables of a class until an object is created | When object is created then memory is allocated to object in heap |
4 | For define the class structure , class keyword is used | For making object , new keyword is used |
5 | Class contains only declarations, no actual data. | Object contains actual data, which are values of instance variables. |
6 | Class contains the method definition. | Object calls the methods to perform the action |
7 | Class is a user-defined data type | Object is an instance of a user-defined data type (class). |
8 | Example : class Rectangle { int length; int width; } | Example : Rectangle rect = new Rectangle(10, 20); |
9 | Class : car | Object : BMW, Toyota, Suzuki, Skoda |
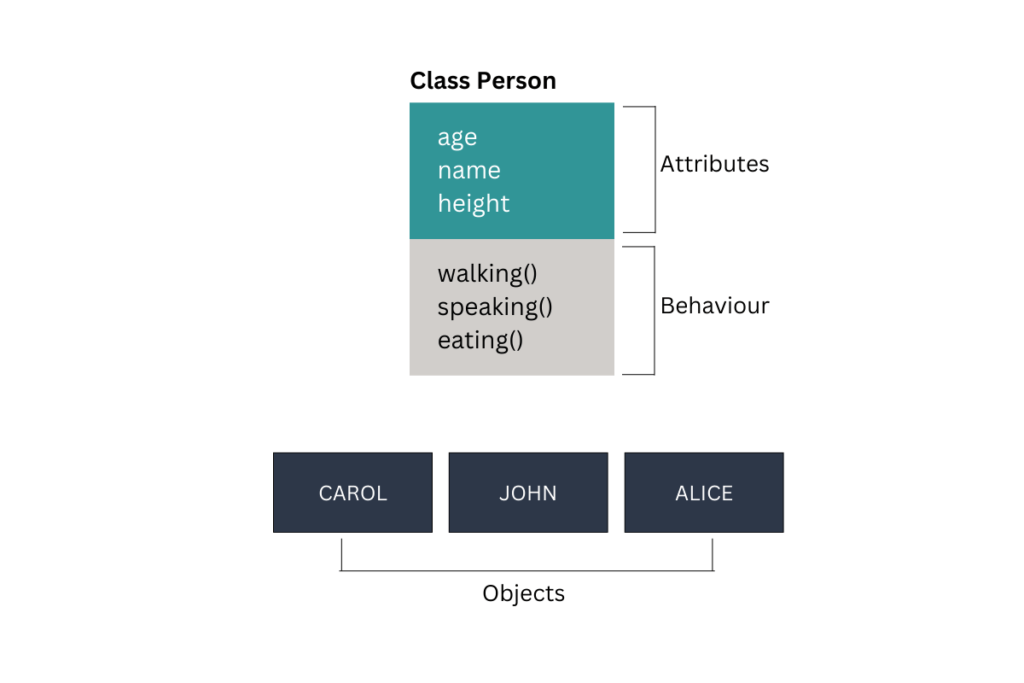
let’s understand the concept of class and object with the help of the program. First, we define the class, and then we make the object of that class
Program Example for showing the Difference Between class and object in Java
// Define a class named Person
class Person {
// Fields (attributes of the class)
String name;
int age;
String phone;
// Method to display the person's details
void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("Phone: " + phone);
}
// New method to check if the person is an adult
boolean isAdult() {
return age >= 18;
}
}
public class Main {
public static void main(String[] args) {
// Create an object of the Person class
Person obj = new Person();
// Assign values to the fields of obj
obj.name = "John";
obj.age = 25;
obj.phone = "833929202";
// Call the displayInfo method to print the person's details
obj.displayInfo();
// Call the new method to check if the person is an adult
if (obj.isAdult()) {
System.out.println(obj.name + " is an adult.");
} else {
System.out.println(obj.name + " is not an adult.");
}
}
}
Explanation:
Person
is the name of the class.- The
Person
class has 3 fields:name
,age
, andphone
, and 2 methods:displayInfo()
andisAdult()
. - An object
obj
is created in themain
method and values are assigned to thename
,age
, andphone
fields ofobj
. - Next, we call the
displayInfo()
method ofobj
, which prints thename
,age
, andphone
ofobj
. - The
displayInfo()
method does not return any value because its return type isvoid
. - After that, we check if
obj
is an adult using theisAdult()
method. - The
isAdult()
method returns a value (true
orfalse
) because its return type isboolean
. - If
isAdult()
returnstrue
, theif
block is executed; otherwise, theelse
block is executed.
Name: John
Age: 25
Phone: 833929202
John is an adult.
Conclusion
The primary difference between classes and objects in Java lies in their nature and purpose. A class is a blueprint that defines the structure and behavior of objects, including fields and methods. In contrast, an object is an instance of a class that holds specific values for those fields. While a class represents a general concept, an object represents a concrete example of that concept in action. Understanding this distinction is crucial for effective object-oriented programming.
We’d Love to Hear From You!
Do you have questions or feedback about the difference between classes and objects in Java? We’re here to help! Whether you need further clarification, have suggestions, or simply want to share your thoughts, please leave a comment below. Your feedback is invaluable in helping us improve our content and support others in their learning journey. Don’t hesitate to reach out—we’re excited to hear from you!
Related Articles:
What Is Class In Java With Example?
What Is an Object in Java?